10 most asked questions about Swift – Programming Language from Apple
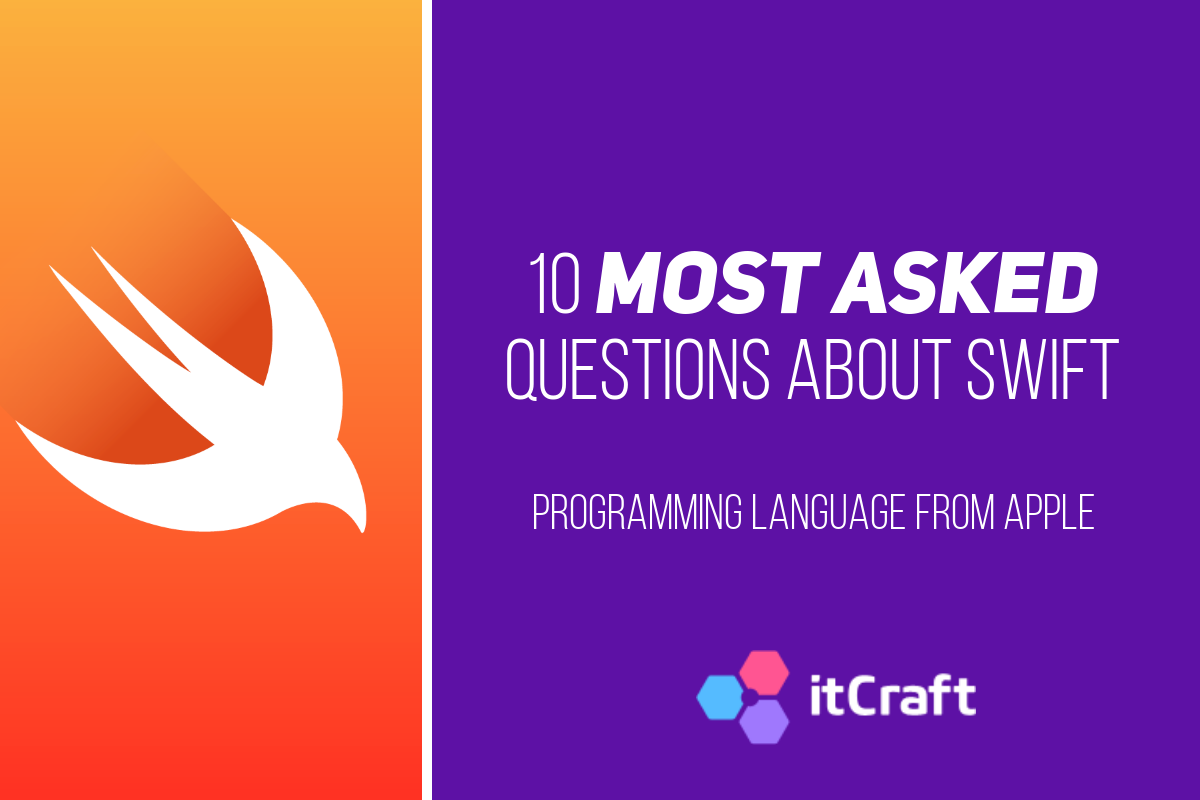
I’d like to give you a quick and easy way to prepare for your next interview for an iOS developer’s job. As you might be aware, the questions programmers get asked are completely different to your standard “Tell me about yourself” “Why should we hire you?”
Most employers or outsourcing companies in the software business are looking for particular skill set and level of proficiency, rather than your general approach to work.
Table of Contents
- What’s the difference between struct and class?
- Does Swift have a package manager similar to npm(js), pip(py), pub(dart)?
– Integrating manually
– Swift package manager
– CocoaPods - How do you convert a double into a string?
- What is an optional value?
- What does “fatal error: unexpectedly found nil while unwrapping an Optional value” mean?
- How to pass data between View Controllers?
- How to add constraints programmatically?
- What is a ‘lazy var’?
- What is an empty string in Swift?
- What are the higher-order functions?
Below, you will find a list of questions (and answers) asked to check your knowledge and understanding of Swift. I learnt these from both experience and research and picked the ones that come up most often.
1. What’s the difference between struct and class?
Structs are value types and classes are reference types. This means that if you point two variables at the same struct, each one will have its own independent copy of the data. Pointing two variables at the same instance of the class, will result in both of them referencing the same object.
Example of a reference type:
class SomeClass {
var classId: Int
init(id: Int) {
self.classId = id
}
}
var classA = SomeClass (id: 10)
var classB = classA // 'classB' references the same object as 'classA'
print(classA.classId)
// prints out '10'
print(classB.classId)
prints out '10'
classA.classId = 20
print(classA.classId)
// prints out '20'
print(classB.classId)
// prints out '20'
Example of a value type:
struct SomeStruct {
var structId: Int
}
var structA = SomeStruct(structId: 10)
var structB = structA // 'structB' gets a new copy of StructA
print(structA.structId)
// prints out '10'
print(structB.structId)
// prints out '10'
structA.structId = 20
print(structA.structId)
// prints out '20'
print(structB.structId)
// prints out '10'
2. Does Swift have a package manager similar to npm(js), pip(py), pub(dart)?
Yes, in fact there are several ways to include third party code in your project :
Integrating manually
This manual process, basically consists of dragging the package into your project and linking it. Pretty easy with few external libraries, but with larger amount of them, maintenance gets more complicated and error-prone.
Swift package manager
Integrated in Swift 3.0 and above, managed by Apple, this manager is the most npm-like from those listed. Since it’s still considered a “new thing” in iOS development, many libraries lack support for this channel of distribution.
This one requires initial manual setup and tends to be slow during the first “build”, but over time it shows its power as following builds will be extremely fast. However, some libraries do not get distributed over Carthage (officially).
CocoaPods
Probably the most popular dependency manager for iOS, very easy to setup and use. Sometimes, it extends the compilation time of your project significantly (e.g with Realm). A lot of libraries are distributed with CocoaPods.
3. How do you convert a double into a string?
You can achieve this in several different ways, as shown in the screenshot below:
import Foundation
let doubleValue = 3.14
print(type (of: doubleValue))
// prints out 'Double'
let stringFromDouble = doubleValue.description
print(type(of: stringFromDouble))
// prints out 'String'
let anotherStringFromDouble = "(doubleValue)"
print(type(of: anotherStringFromDouble))
// prints out 'String'
let yetAnotherStringFromDouble = "(doubleValue)"
print(type(of: yetAnotherStringFromDouble))
// prints out 'String
4. What is an optional value?
Optional variable can have one of two states, it either contains a value or it is ‘nil’ (no given value). In Swift, you usually declare optional types with a question mark (e.g Int?, String?, Double?). In order to use the value stored in the optional variable, you need to unwrap it using: optional binding, optional chaining, force unwrapping or nil-coalescing operator.
5. What does “fatal error: unexpectedly found nil while unwrapping an Optional value” mean?
This runtime error is typically being triggered whenever you access the optional value that is ‘nil’ in a non-safe manner (using force unwrapping or implicitly unwrapped optionals).
6. How to pass data between View Controllers?
There are many ways to achieve this, e.g: segues, properties, functions, delegates, closures, Notification Center. Some of them are used more often, others less so. It’s worth learning how each of them works and where to apply a specific pattern. As this is a extensive topic, it would require a separate article to cover. I suggest the link below.
7. How to add constraints programmatically?
You can either use one of Apple’s recommended ways: NSLayoutConstraint, NSLayoutAnchor or Visual Format Language
Click here for more info
or use a third party library like SnapKit, which is a domain-specific language (DSL) for making AutoLayout simple and expressive
8. What is a ‘lazy var’?
A ‘lazy stored property’ is a property with the initial value calculated on its first use. It is very useful when you want to perform some performance-heavy calculations under certain conditions (e.g triggered by a specific event).
9. What is an empty string in Swift?
According to the documentation for ‘isEmpty’ property, a string is considered empty when it has no characters.
10. What are the higher-order functions?
These functions let you make operations on the sequence in a very elegant manner, e.g:
- map(_:) – iterates through each element of the sequence, transforms it based on a closure passed to the function, and returns a new sequence with transformed elements,
- reduce(_:_:) – returns the result of combining the elements of the sequence using the given closure,
- filter(_:) – returns a new sequence with elements that satisfy the criteria defined in a closure passed to the method,
Click here for more info