MVC MVP and MVVM architecture pattern – Introduction
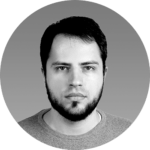
Michał Trętowicz
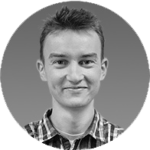
Adrian Zalewski
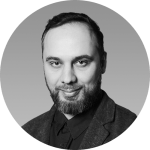
Jakub Turkowski
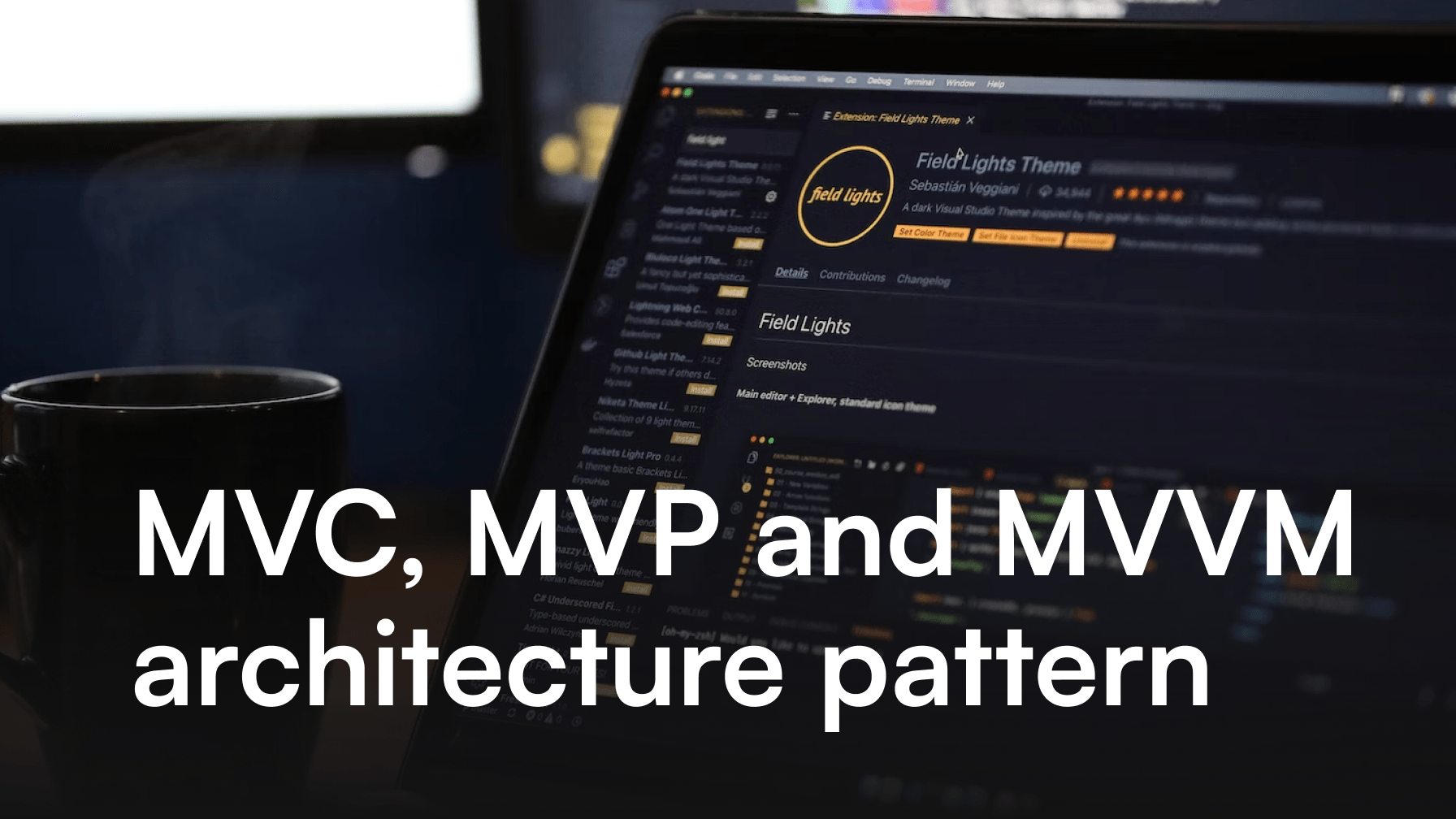
Let’s go deep into the Android app architecture. There are three layers in each of described architectural patterns. View layers which receives user input. Then there’s a model which describes data used in your application. Finally we have a bridge between model and view layers which is data presentation logic. The main goal of using architecture pattern is to separate concerns.
Table of contents
1. MVC (model view controller)
2. MVP (model view presenter) architecture pattern
3. MVVM architecture (model view viewmodel)
4. MVC vs MVP vs MVVM – A brief summary of architecture patterns
MVC (model view controller)
MVC pattern – design pattern facilitates the organization of the application structure thanks to the division into three layers: View, Controller and Model. Let’s go through each of the pattern layers in more detail.
Model view controller – about the model
Model is the part of the code responsible for contact with data sources. It is an intermediary between the data source and the view. It deals with storing data and reading the data, as well as ensuring their consistency and validation. The model contains the entire business logic of the application. Thanks to the separation of responsibilities, the code becomes more structured and flexible to modifications (changing one layer does not necessarily entail changing the code in the other layers).
MVC design pattern – view (ui logic)
The view is the part of the code responsible for the presentation of the data retrieved from the model to the user. The view does not have any business logic, it focuses only on displaying the data, which facilitates subsequent changes in the application. A view can change the state of the model only if the modification involves a change in the way data is displayed. If we want to change the appearance of the application, we do not have to interfere with the code responsible for the logic. So, UI components that are changed (in terms of updated data) have an impact on view interface for user, but don’t have an impact on the application logic code.
Controller
The controller handles the interaction with the user. Contains core business logic for handling events passed from the view. The controller allows access to certain parts of the application only by authorized users. Depending on the user’s actions, it updates the model and refreshes the view. It can also transfer control to another controller.
Benefits
- Division into modules organizing the application code,
- Separation of business logic from view,
- The model is not dependent on the view,
- Makes it easier to find a specific part of the code,
- Easier expansion through modular construction,
- Prevents clutter in the code,
- Facilitates teamwork.
Disadvantages
- Complicated division into modules increasing the complexity of the system,
- Due to the specificity of the Android system architecture (life and activity cycle), the View and Controller layers are often implemented in an activity, a fragment, so there is no clear separation between them (therefore only the model layer is able to perform unit testing),
- Dependence of the view on the model,
- Difficult to test views,
- The pattern can be used in small projects with a small number of screens, where, if necessary, the business logic is tested.
MVP (model view presenter) architecture pattern
The MVP is an architecture pattern that improves the process of creating application screens by dividing responsibilities into three layers: view, presenter and model. MVP was created on the basis of the MVC pattern. In the model view presenter pattern, the presenter is the same as the controller in MVC with one small difference, there is the business logic in the presenter. Data is not passed directly from the model to the view as it is in MVC. The presenter queries the model, the model returns the data to the presenter, the presenter processes the received data and passes it to the view.
Model
The model represents the part of the code that is responsible for managing business logic and supporting network or database API (works with the remote and local data sources to get and save the data). It also definesdomain logic (business rules) for data means how the data can be changed and manipulated.
View (user interface)
The view in the MVP design pattern is the part of the code responsible for the presentation of the data provided by the presenter to the user. The view does not have any business logic, it focuses only on displaying the data, which facilitates subsequent changes in the application. If we want to change the appearance of the application, we do not have to interfere with the code responsible for the logic. When user interacts with UI presenter logic is fired.
Presenter (business logic)
The presenter contains all the business logic of the application and handles the user interactions. It’s presenting data to the view layer. Depending on actions of the user, it reads the necessary data through the model, performs the necessary calculations and passes the result of its calculations to the view. The presenter also handles user authorizations and authentication, and allows only authorized users to access specific parts of the application.
Benefits
- Division into modules organizing the application code,
- Separation of business logic from view,
- The model is not dependent on the view,
- Makes it easier to find a specific part of the code,
- Easier expansion through modular construction,
- Facilitates teamwork,
- Is used to solve the problem of large activities, fragments (which have too much responsibility and a lot of code).
Disadvantages
- Due to the lifecycle of a fragment or activity, or other user interface components, a reference memory leak may appear for a view that does not exist anymore in the presenter. In such a case, care should be taken to adequately support lifecycle methods,
- It often happens that the implementation of a pattern requires the creation of additional classes and interfaces (often with similar content), which is often associated with redundant code,
- Too complicated for simple, small applications,
- Requires familiarizing employees with the pattern, which results in higher costs,
- Presenter has a reference to the view so if we want to use it elsewhere we need to implement that view in for example fragment. This can result in many unnecessary empty functions implemented,
- Tight coupling between presenter and view (one to one relationship).
MVVM architecture (model view viewmodel)
MVVM design pattern that is designed to facilitate the creation of application screens by using the division of responsibilities into three different layers: view, model view (ViewModel) and model (Model). The idea of the MVVM pattern is based primarily on the view layer (Observer pattern) observing the changing data in the model view layer and responding to changes through the data binding mechanism. Thanks to the use of data binding strategy in the view layer, its logic is minimized, the code becomes more structured and open to modifications, and testing is easier.
It’s great choice for small scale projects. It also works great with two way data binding. It’s event driven (means view reacts to state changes in View model). Because view model doesn’t have reference to the view layer unit testing is easier. Major components of MVVM are provided by Google. Main three components are: Activity or fragment containing the view model, view model and network and database repositories.
Model (business logic)
The Model is responsible for the business logic, i.e. processing, storage, modification and delivery of the expected data to the ViewModel.
Design pattern – View (user interface)
The View layer is responsible for the presentation of data, system state and current operations in the graphical interface, as well as for the initialization and binding of the ViewModel to the view elements. Here we setup user input interaction. It is an entry point of user interaction.
ViewModel
Deals with delivering data from the Model to the View layer and handling user actions. It is worth mentioning that it provides the data streams for View. Think of it as a bridge between the Model and View. When Model changes ViewModel provides update of data state to view component through mechanism called LiveData (observable variables). One view may contain many different view models (one to many relationship).
Benefits
- Solves the memory leak problem that appears in the MVP pattern (the model view does not have a reference to the view layer),
- No duplication of data,
- Independence of logic from the way data is displayed,
- Thanks to the separation of tasks in different layers, the pattern can be used to increase the readability of the code and the possibility of independent testing without the use of additional dependencies,
- No interfaces defining views like in MVP,
- The data binding mechanism increases the flexibility of the view on modifications, which makes the MVVM pattern work well in large dynamically changing projects.
Disadvantages
- Each view screen has a dedicated ViewModel, which translates into a greater number of classes (compared to the MVP, there are still fewer of them),
- The view layer must ensure proper binding of variables and methods for each required view element, as well as observing the state of the ViewModel,
- Creating a correct and complete ViewModel requires analyzing the view layer in terms of the required data and possible states,
- Proper handling of LiveData (live events from viewModel to view layer) requires more boilerplate code,
- In some cases data binding mechanism can get complex. You need to be careful when you modify your UI layout files,
- When used with data binding you need to be aware of clearing binding every time user leaves the screen, otherwise memory leaks might occur.
MVC vs MVP vs MVVM
A brief summary of architecture patterns
In MVC the Controller handles events, manipulates the Model, which contains business logic, and the View displays data from the Model. One Controller supports several Views.
In MVP, the data from the Model is passed to the Presenter and not directly to the View, and the Presenter passes it to the View. One presenter can refer to one View.
In MVVM, the data from the Model is passed to the ViewModel, which can support multiple Views. The View knows nothing about the ViewModel, it only requires the data you need.
Which architectural pattern is the best?
We have to start thinking about what’s the goal of all these mentioned architectures. The goal is always the same – make your code readable, more maintainable, more testable and just easier to work on with a team. No matter what kind of pattern you look at, every single pattern has the same goal and wants to achieve the same things. The foundation of good software architecture will always be the same few things like: modular design, like SOLID principles, writing proper abstractions, Single Source of Truth, a single responsibility principle, separation of concerns – all these things are important for any type of architecture.
Architecture pattern – flexibility pays off
No matter what kind of pattern you use, you don’t need to stick to a specific different kind of pattern by a hundred percent, because you understand what makes an architecture good and you can still deviate from that a little bit and still have a good testable architecture. That should be the goal. It is worth to mention that MVVM pattern is supported directly by Google so you don’t have to use third party libraries or build architectural patterns by yourself. It’s also recommended by Google and widely accepted by many other developers so it’s common that this is a first choice for architectural pattern when developing new application.
Remember that in all of the presented architectural patterns we can easily separate database and network layers making development and maintenance so much easier. There are many design patterns not covered in this article but keep in mind that MVP and MVVM are the most popular ones, so you can get really great support from the android developers community while using them.